- Deployment controller provides declarative updates for Pods and it manages stateless applications running on your cluster.
- Deployments represent a set of multiple, identical Pods and upgrade them in a controlled way, performing a rolling update by default.
- A Deployment runs multiple replicas of your application and automatically replaces any instances that fail or become unresponsive.
- In this way, Deployments ensure that one or more instances of your application are available to serve user requests.

Create a deployment Yaml File:
Follow the following statute and copy the pod template from the nginx-pod that you have created before.
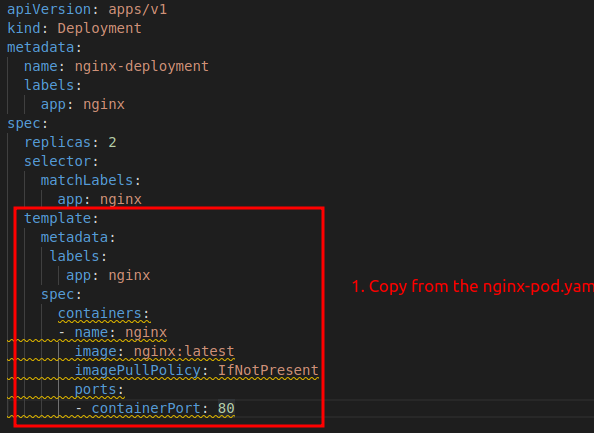
Note: Deployment file writing tips are at the end of this deployment section, so do learn more.
$ sudo vim nginx-deployment.yaml |
Enter the following manifest
apiVersion: apps/v1 kind: Deployment metadata: name: nginx-deployment labels: app: nginx spec: replicas: 2 selector: matchLabels: app: nginx template: metadata: labels: app: nginx spec: containers: – name: nginx image: nginx:latest imagePullPolicy: IfNotPresent ports: – containerPort: 80 |
Let’s Create the nginx deployment:
$ kubectl create -f nginx-deployment.yaml |
deployment.apps “nginx-deployment” created
Display your deployments:
$ kubectl get deployments |
Expected Output:
NAME READY UP-TO-DATE AVAILABLE AGE
nginx-deployment 2/2 2 2 10m
Get details of a deployment:
$ kubectl describe deployment nginx-deployment |
Check the Pods:
$ kubectl get pods |
Expected Output:
NAME READY STATUS RESTARTS AGE
nginx-deployment-54b6f7ddf9-t9vct 1/1 Running 0 12m
nginx-deployment-54b6f7ddf9-z77r2 1/1 Running 0 12m
Check the status of the deployment:
Note: Kubernetes deployment rollout is the process of updating or replacing existing pods in a Kubernetes deployment. It is a crucial part of managing containerized applications in a Kubernetes environment. The rollout process can be performed using the “kubectl rollout” command or through the Kubernetes API. There are various strategies for deployment rollout, including blue-green and canary deployments. It’s essential to understand the rollout process and plan it carefully to minimize downtime and ensure high availability of applications.
$ kubectl rollout status deployment/nginx-deployment |
deployment “nginx-deployment” successfully rolled out
Update the deployment:
$ kubectl set image deployment/nginx-deployment nginx=nginx:latest |
deployment.apps “nginx-deployment” image updated
Rollback to previous revision:
$ kubectl rollout undo deployment/nginx-deployment |
deployment.apps “nginx-deployment”
$ kubectl rollout status deployment/nginx-deployment |
deployment “nginx-deployment” successfully rolled out
Check Rollout history:
$ kubectl rollout history deployment/nginx-deployment |
deployments “nginx-deployment”
REVISION CHANGE-CAUSE
2 < none >
3 < none >
Scale a deployment:
$ kubectl scale deployment/nginx-deployment –replicas=5 |
deployment.apps “nginx-deployment” scaled
$ kubectl get pods |
NAME READY STATUS RESTARTS AGE
nginx-deployment-54b6f7ddf9-6glt7 1/1 Running 0 4s
nginx-deployment-54b6f7ddf9-89bdn 1/1 Running 0 2m8s
nginx-deployment-54b6f7ddf9-kx75w 1/1 Running 0 2m9s
nginx-deployment-54b6f7ddf9-v49xn 1/1 Running 0 4s
nginx-deployment-54b6f7ddf9-v6b7j 1/1 Running 0 4s
Edit a deployment:
$ kubectl edit deployment nginx-deployment |
Delete a deployment:
$ kubectl delete deployment nginx-deployment |
Writing a Deployment Spec
A Deployment manifest needs
- apiVersion
- kind
- metadata
- spec
fields. The metadata field has names, labels, annotations, and other information.
The spec field has replicas, deployment strategy, pod template, selector, and other details.
Pod Template
The spec.template is the only required field of the .spec.
The spec.template is a pod template. It has the same schema as a Pod, except it is nested and does not have an apiVersion or kind.
spec: template: metadata: labels: app: frontend |
Restart Policy
Only a spec.template.spec.restartPolicy equal to Always is allowed, which is the default if not specified.
spec: template: metadata: labels: app: frontend spec: restartPolicy : Always containers: |
Replicas
spec.replicas is an optional field that specifies the number of desired Pods. It defaults to 1.
spec: replicas: 3 |
Selector
spec.selector is an optional field that specifies a label selector for the Pods targeted by this deployment.
spec: replicas: 3 selector: matchLabels: app: fronted |
spec.selector must match .spec.template .metadata.labels, or it will be rejected by the API.
spec: replicas: 3 selector: matchLabels: app: fronted template: metadata: labels: app: fronted |
Strategy
spec.strategy specifies the strategy used to replace old Pods by new ones. spec.strategy.type can be “Recreate” or “RollingUpdate”. “RollingUpdate” is the default value.
spec: replicas: 3 strategy: type: RollingUpdate |
Deployment Failure
Your Deployment may get stuck trying to deploy its newest ReplicaSet without ever completing it.
This can occur due to some of the following factors:
- Insufficient quota
- Readiness probe failures
- Image pull errors
- Insufficient permissions
- Limit ranges
- Application run-time misconfiguration